I have been looking at an site with a custom post type to advertise jobs and custom fields to represent all the fields that need to be filled in by the site admin.
One of the fields is an expiry date that is set using the ACF (Advanced Custom Fields) date picker. What I wanted to do was disable the application form on the job at the expiry date, without having to have a custom form for every job.
There are various plugins to do things like this, but in my case they didn’t seem to work that well and a few hadn’t been updated in a long time. However, it turns out that as this is to go in the template, rather than on the WordPress page, it’s actually pretty easy to write in PHP and just include that in the template.
The code snippet follows: –
<?php
// For US format dates use $date1 = date("m/d/y")
$date1 = date("d-m-Y");
$closing1 = get_field('closing_date');
$date2 = strtotime($date1);
$expires1 = strtotime($closing1);
if ($date2 < $expires1) {
echo "<p>Todays Date - " . $date1 ."</p>";
echo "<p>This will expire on " . $closing1 . "</p>";
} else {
echo "<p>Todays Date - " . $date1 ."</p>";
echo "<p>This expired on " . $closing1 . "</p>";
}
?>
To explain this, the first line sets the variable $date1 as the current date from the server. Note the date format is d-m-Y. This is the standard for European format dates as per https://www.php.net/manual/en/datetime.formats.php but for US format you will want m/d/y. Note the different order and slashes not dashes.
The second line sets a variable of $closing1 which retrieves my ACF field closing_date. Note that the ACF field has to be set up to the same date format or you will need to do some extra php work get it into this format. My ACF field settings look like the below: –
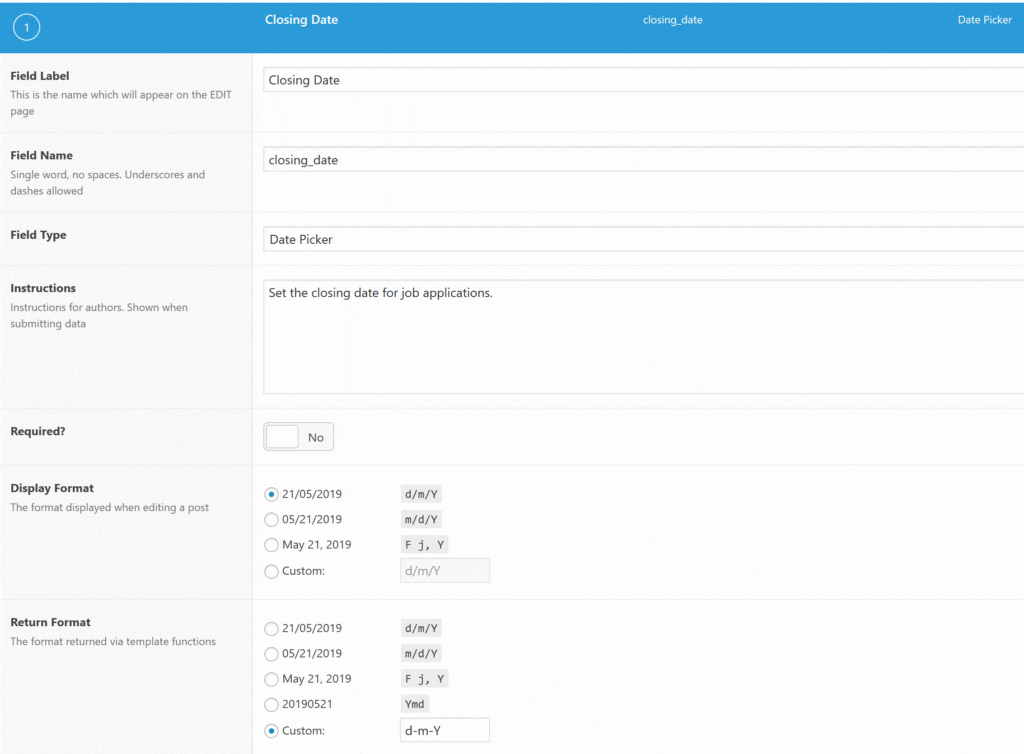
Once you have these variables set, that is most of the hard work done.
Lines 3 and 4 take the above 2 variables and convert them into a standard form the server will understand and be able to work with (the number of seconds since January 1 1970 00:00:00 UTC).
After that we have a simple if statement comparing the 2 times and customising content to suit. In this case if todays date is less than the expiry date show the first content, if it’s after show the second content. Just substitute in what you need.
In my case I am going to put the Gravity Forms shortcode in the top section, so the application for will show if the expiry hasn’t past. In the bottom section I will put a message saying the the expiry date has passed, but if they want to contact HR, the details are shown.
Next step will be to move the job to draft a couple of days after the expiry date!